<?php $str = <<<'EOT' {"name_first":"John"} EOT; echo json_encode(json_decode($str), JSON_PRETTY_PRINT | JSON_UNESCAPED_SLASHES);^ Save the file. Name it something such as json-pretty-printer.php and then run it on the command line by typing 'php' then the path to your script. Hit enter. Boom.
One Q, One A
Friday, December 2, 2016
How Do I Make a JSON Pretty Printer App Using PHP for Development/Debugging Use as a Coder?
Ya'll know you don't wanna be posting your app's JSON responses, which may include sensitive information, to some third party website which could easily or accidentally log this info... think, decrypted passwords might be in your response, API keys, the name of your app. Lot's of stuff ain't nobody but you should see. So here, make your own JSON PRETTY PRINTER using PHP and RUN IT LOCALLY on your comp. Yea boi! Get it!
Saturday, January 30, 2016
Wednesday, January 27, 2016
How can I search the entire (unix) file system for files whose name contains a specific substring?
# To list files whose name contains "yoursearchterm" find / -name "*yoursearchterm*" -printSwap the "/" with a more specific path to limit your search. Add "-maxdepth 1" after the path and before "-name" to limit your search to a single directory.
Credit: http://stackoverflow.com/a/11329078
Friday, July 17, 2015
How to link your Mac's standard php location (/usr/bin/php) to a specific version somewhere else in the file system.
To map your Mac's standard php location to a specific version somewhere else in the file system, run the following command:
sudo ln -s /Applications/MAMP/bin/php/php5.5.3/bin/php /usr/bin/php
Wednesday, April 15, 2015
How Do I Configure iTunes to Store iOS Device Backups on an External Hard Drive?
Sadly, this is done via Terminal rather than iTunes.
ln -s /Volumes/1TB\ Fantom/iOSDeviceBackups/Backup /Users/johnerck/Library/Application\ Support/MobileSync/
Monday, December 15, 2014
Thoughts on setting up a CentOS 7 box
# VARIABLES: # my_ip_address: 23.253.55.25 # my_first_not_root_user: admin # my_ssh_port: 4972 # my_bitbucket_project_1_owner_username: abovemarket # my_bitbucket_project_1_name: logs.abovemarket.com # my_bitbucket_project_2_owner_username: abovemarket # my_bitbucket_project_2_name: new.abovemarket.com # my_server_admin_email_address: john.erck@abovemarket.com # my_local_path_to_wildcard_crt: ~/Business/Above\ Market/SSL/STAR_abovemarket_com/STAR_abovemarket_com.crt # my_local_path_to_wildcard_ca_bundle: ~/Business/Above\ Market/SSL/STAR_abovemarket_com/STAR_abovemarket_com.ca-bundle # my_local_path_to_wildcard_pem: ~/Business/Above\ Market/SSL/STAR_abovemarket_com.pem # my_local_path_to_wildcard_key: ~/Business/Above\ Market/SSL/STAR_abovemarket_com.key # my_remote_filename_for_wildcard_crt: STAR_abovemarket_com.crt # my_remote_filename_for_ca_bundle: STAR_abovemarket_com.ca-bundle # my_remote_filename_for_pem: STAR_abovemarket_com.pem # my_remote_filename_for_key: STAR_abovemarket_com.key # Create new CentOS 7 box, then: ssh root@my_ip_address passwd useradd my_first_not_root_user passwd my_first_not_root_user visudo # Add "my_first_not_root_user ALL=(ALL) ALL" after "root" nano /etc/ssh/sshd_config # Update "Port" to my_ssh_port systemctl restart sshd.service vim myfirewall # myfirewall TEMPLATE TEXT OPEN #!/bin/bash # # iptables example configuration script # # Flush all current rules from iptables # iptables -F # # Allows all loopback (lo0) traffic and drop all traffic to 127/8 that doesn't use lo0 iptables -A INPUT -i lo -j ACCEPT iptables -A INPUT ! -i lo -d 127.0.0.0/8 -j REJECT # # # Accepts all established inbound connections iptables -A INPUT -m state --state ESTABLISHED,RELATED -j ACCEPT # # # Allows all outbound traffic # You can modify this to only allow certain traffic iptables -A OUTPUT -j ACCEPT # # # Allows HTTP and HTTPS connections from anywhere (the normal ports for websites) iptables -A INPUT -p tcp --dport 80 -j ACCEPT iptables -A INPUT -p tcp --dport 443 -j ACCEPT # # # Allows SSH connections # # THE -dport NUMBER IS THE SAME ONE YOU SET UP IN THE SSHD_CONFIG FILE # iptables -A INPUT -p tcp -m state --state NEW --dport my_ssh_port -j ACCEPT # # # Allow ping iptables -A INPUT -p icmp -m icmp --icmp-type 8 -j ACCEPT # # # log iptables denied calls iptables -A INPUT -m limit --limit 5/min -j LOG --log-prefix "iptables denied: " --log-level 7 # # # Reject all other inbound - default deny unless explicitly allowed policy iptables -A INPUT -j REJECT iptables -A FORWARD -j REJECT # # # Save settings # /sbin/service iptables save # # List rules # iptables -L -v # # myfirewall TEMPLATE TEXT CLOSE chmod +x myfirewall ./myfirewall yum update yum install httpd # Apache yum install mysql # For release purposes needed on app server yum install php php-mysql # The mother ship yum install php-gd # Needed for app server image processing functions to work yum install git yum install mod_ssl openssl systemctl enable httpd.service # So that it will automatically start after a reboot exit scp -P my_ssh_port ~/.ssh/id_rsa.pub root@my_ip_address:my_machine_id_rsa.pub ssh -p my_ssh_port root@my_ip_address cat my_machine_id_rsa.pub >> ~/.ssh/authorized_keys chmod 700 ~/.ssh chmod 600 ~/.ssh/authorized_keys restorecon -Rv ~/.ssh # Ensure the correct SELinux contexts are set exit scp -P my_ssh_port ~/.ssh/id_rsa.pub admin@my_ip_address:my_machine_id_rsa.pub ssh -p my_ssh_port admin@my_ip_address cat my_machine_id_rsa.pub >> ~/.ssh/authorized_keys chmod 700 ~/.ssh chmod 600 ~/.ssh/authorized_keys restorecon -Rv ~/.ssh # Ensure the correct SELinux contexts are set ssh-keygen -t rsa -C "my_server_admin_email_address" cat /home/my_first_not_root_user/.ssh/id_rsa.pub # Then, go to: https://bitbucket.org/my_bitbucket_project_1_owner_username/my_bitbucket_project_1_name/admin/deploy-keys # and add the key as "my_first_not_root_user@my_ip_address" # Then, go to: https://bitbucket.org/my_bitbucket_project_2_owner_username/my_bitbucket_project_2_name/admin/deploy-keys # and add the key as "my_first_not_root_user@my_ip_address" exit # Copy your SSL certificate file and the certificate bundle file to your Apache server. # You should already have a key file on the server from when you generated your certificate # request. If not, transfer that too. scp -P my_ssh_port my_local_path_to_wildcard_crt root@my_ip_address:my_remote_filename_for_wildcard_crt scp -P my_ssh_port my_local_path_to_wildcard_ca_bundle root@my_ip_address:my_remote_filename_for_ca_bundle scp -P my_ssh_port my_local_path_to_wildcard_pem root@my_ip_address:my_remote_filename_for_pem scp -P my_ssh_port my_local_path_to_wildcard_key root@my_ip_address:my_remote_filename_for_key ssh -p my_ssh_port root@my_ip_address mv my_remote_filename_for_wildcard_crt /etc/pki/tls/certs/my_remote_filename_for_wildcard_crt mv my_remote_filename_for_ca_bundle /etc/pki/tls/certs/my_remote_filename_for_ca_bundle mv my_remote_filename_for_pem /etc/pki/tls/private/my_remote_filename_for_pem mv my_remote_filename_for_key /etc/pki/tls/private/my_remote_filename_for_key vim +/SSLCertificateFile /etc/httpd/conf.d/ssl.conf # Update file like so: SSLCertificateFile /etc/pki/tls/certs/my_remote_filename_for_wildcard_crt SSLCertificateKeyFile /etc/pki/tls/private/my_remote_filename_for_key SSLCACertificateFile /etc/pki/tls/certs/my_remote_filename_for_ca_bundle systemctl restart httpd.service mkdir -p /home/admin/my_bitbucket_project_1_name mkdir -p /home/admin/my_bitbucket_project_2_name chown -R my_first_not_root_user:my_first_not_root_user /home/admin/my_bitbucket_project_1_name chown -R my_first_not_root_user:my_first_not_root_user /home/admin/my_bitbucket_project_2_name su my_first_not_root_user cd /home/admin/my_bitbucket_project_1_name git clone git@bitbucket.org:my_bitbucket_project_1_owner_username/my_bitbucket_project_1_name.git . cd /home/admin/my_bitbucket_project_2_name git clone git@bitbucket.org:my_bitbucket_project_2_owner_username/my_bitbucket_project_2_name.git . exit mkdir /etc/httpd/sites-available mkdir /etc/httpd/sites-enabled vim /etc/httpd/conf/httpd.conf # Add the following line to the end of the file: IncludeOptional sites-enabled/*.conf vim /etc/httpd/sites-available/my_bitbucket_project_1_name.conf # Add the following text: <VirtualHost *:80> ServerName www.my_bitbucket_project_1_name ServerAlias my_bitbucket_project_1_name DocumentRoot /home/admin/my_bitbucket_project_1_name/www ErrorLog /home/admin/my_bitbucket_project_1_name_error.log CustomLog /home/admin/my_bitbucket_project_1_name_requests.log combined </VirtualHost> <VirtualHost *:443> ServerName www.my_bitbucket_project_1_name ServerAlias my_bitbucket_project_1_name DocumentRoot /home/admin/my_bitbucket_project_1_name/www ErrorLog /home/admin/my_bitbucket_project_1_name_error.log CustomLog /home/admin/my_bitbucket_project_1_name_requests.log combined </VirtualHost> vim /etc/httpd/sites-available/my_bitbucket_project_2_name.conf # Add the following text: <VirtualHost *:80> ServerName www.my_bitbucket_project_2_name ServerAlias my_bitbucket_project_2_name DocumentRoot /home/admin/my_bitbucket_project_2_name/www ErrorLog /home/admin/my_bitbucket_project_2_name_error.log CustomLog /home/admin/my_bitbucket_project_2_name_requests.log combined </VirtualHost> <VirtualHost *:443> ServerName www.my_bitbucket_project_2_name ServerAlias my_bitbucket_project_2_name DocumentRoot /home/admin/my_bitbucket_project_2_name/www ErrorLog /home/admin/my_bitbucket_project_2_name_error.log CustomLog /home/admin/my_bitbucket_project_2_name_requests.log combined </VirtualHost> ln -s /etc/httpd/sites-available/my_bitbucket_project_1_name.conf /etc/httpd/sites-enabled/my_bitbucket_project_1_name.conf ln -s /etc/httpd/sites-available/my_bitbucket_project_2_name.conf /etc/httpd/sites-enabled/my_bitbucket_project_2_name.conf apachectl restart # Make sure you've mapped your DNS records to point to my_ip_address for each of # the project names/domains you setup. # Boom, you're done.
Thursday, October 9, 2014
How does one go about creating a softlink?
ln -s ~/Dropbox/secure/project/_development.yaml clitools/_development.yaml ln -s ~/Dropbox/secure/project/_local.yaml clitools/_local.yaml ln -s ~/Dropbox/secure/project/_production.yaml clitools/_production.yaml ln -s ~/Dropbox/secure/project/_release.yaml clitools/_release.yaml
Subscribe to:
Posts (Atom)
About Me
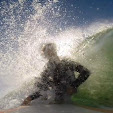
- John Erck
- I code. I figured I should start a blog that keeps track of the many questions and answers that are asked and answered along the way. The name of my blog is "One Q, One A". The name describes the format. When searching for an answer to a problem, I typically have to visit more than one site to get enough information to solve the issue at hand. I always end up on stackoverflow.com, quora.com, random blogs, etc before the answer is obtained. In my blog, each post will consist of one question and one answer. All the noise encountered along the way will be omitted.